Welcome back everyone in this lecture we’re now finally going to discuss how to write your own Python modules and packages.
Let’s explore how to career own modules and packages and to clarify some of the semantics here.
Python Modules
Python modules are really just a .py script that you call in another .py script. A Python module to be the same as the code library.
Creating a Module:
To create a module we have to just save the code in a file with the file extension .py
:
Example:
Save this code in a form of file and named as mymodule.py
.
def mymodule(name):
print("Hello, " + name)
How to Use a Module in python?
For using the modules in python we are using import statement.
Syntax: module_name.function_name.
For example: earlier we have created our own module mymodule now we are going to use that.
import mymodule
mymodule.mymodule("Jon")
How to re-name a module?
We can create an alias when we import a module, by using the as
keyword:
For example: We can create an alias for mymodule
called ma
:
import mymodule as ma
a = ma.person1["name"]
print(a)
Output will be: Jon
What is PYTHONPATH in Python Module?
PYTHONPATH is an environment variable with a list of directories. It uses to set the path for the user-defined python modules. So that it can be straightly imported into a Python program.
Python Built-in Modules
Python have various built-in modules which we can use in our program. For example:
import math
print("The value of pi is:", math.pi)
Output: The value of pi is: 3.141592653589793
To display a list of all available modules, use the following command in the Python console:
>>>help('modules')
The dir() Function
The dir() built-in function returns a sorted list of strings containing the names defined by a module.
By using this function we can see the list of the names of all the modules, variables, and functions that are defined in a module. For example −
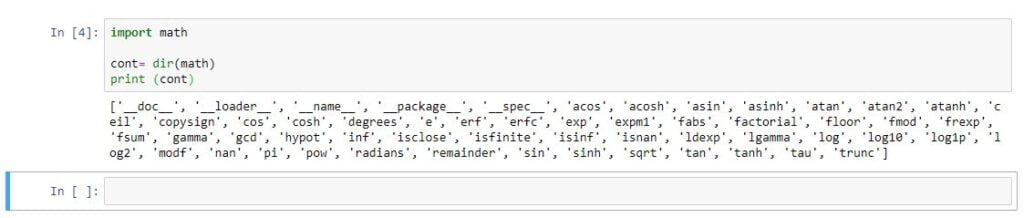
Package in Python:
Python packages are then a collection of modules. Or we can say that the Python application environment consists of modules and sub-packages and subpackages, and so on.
Importing module from a package
A Python package is a directory with Python files and a file with the name __init__
.py. It means that every directory inside the Python path, that contains a file named __init__
.py, and will be treated as a python package.
If we create a directory called calc, that marks the package name, then create a module inside that package called sum
. We should also not forget to add the __init__.py
file inside the calc
directory.
To use the module sum , you can import it in two ways:
1. import calc.sum
OR
2. from calc import sum
In the first method, we can use import a package in the python module by using dot(.), and in the 2nd method, you can import the package sum from calc python modules.
FAQs:
How will we convert a string to a frozen set in python?
we can convert a string to a frozen set by the following command.
frozenset(s) −It Converts string to a frozen set.
What is work of isdigit() in Python?
To check that a string that all characters are digits or not.It will return the True and False.
A Python module contains ………………………
A Python module contains arrays, dictionaries, objects etc.
What is the output of −33 == 33.0?
True
How to create a Python frame?
Frame = Frame()
Must read:
That’s the basic idea of packages and modules. If you have any questions feel free to post your queries and we’ll be happy to help you out there.
I’ll see you at the next post..