Hello everyone and welcome to the Numpy Array Index. In this lecture, We’re going to discuss how to select elements or group elements from a NumPy.
All right let’s go ahead and jump to the Jupiter notebook to get start the Numpy Array Index:
Here, we are going to do only practical examples of python NumPy array indexing with some descriptions, if you want more theory or documentation on this then click here which redirect you to Numpy tutorials official site
You know what I’m going to start off with is by creating a sample array I’m going to say the variable
import numpy as np
#Creating sample array
arr = np.arange(0,11)
#Show
arr
Output: array([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
Bracket Indexing and Selection
The simplest way to pick one or some elements of an array that looks very similar to any python list is mentioned below:
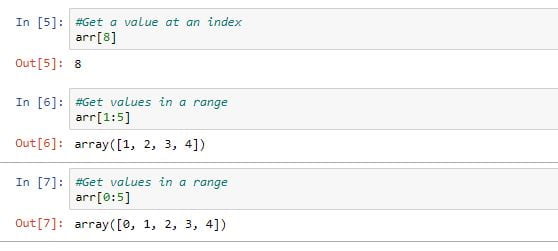
Broadcasting
In Python, Numpy arrays differ from a normal Python list because of their ability to broadcast:
#Setting a value with index range (Broadcasting)
arr[0:5]=100
#Show
arr
Output: array([100, 100, 100, 100, 100, 5, 6, 7, 8, 9, 10])
# Reset array, we'll see why I had to reset in a moment
arr = np.arange(0,11)
#Show
arr
Output: array([ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
Here, some operation of slicing on NumPy array:

Indexing a 2D array (matrices)
The general format is arr_2d[row][col] or arr_2d[row,col]. I recommend usually using the comma notation for clarity.
arr_2d = np.array(([5,10,15],[20,25,30],[35,40,45]))
#Show
arr_2d
Output:
array([[ 5, 10, 15],
[20, 25, 30],
[35, 40, 45]])
#Indexing row
arr_2d[1]
Output: array([20, 25, 30])
# 2D array slicing
#Shape (2,2) from top right corner
arr_2d[:2,1:]
Output:
array([[10, 15],
[25, 30]])
Fancy Indexing in Numpy Array Index
Fancy indexing allows you to select entire rows or columns out of order, to show this, let’s quickly build out a NumPy array:
#Set up matrix
arr2d = np.zeros((10,10))
#Length of array
arr_length = arr2d.shape[1]
#Set up array
for i in range(arr_length):
arr2d[i] = i
arr2d
Output:

some more examples on fancy indexing:

This is all about the Numpy array index with some code examples. I hope you like it. Don’t forget to read the previous articles on the NumPy tutorial.
FAQs:
What is use of numpy.itemsize?
Ans. This array attribute returns the length of each element in bytes
Explain immutability?
Ans. Once an object is created you will not be able to modify the elements, which refers to immutability.
How to access two columns from an array at a time ?
Ans. To access the columns from the array please use the below code
a[:,0:2] —> to access first and 2nd column
Why are we using axis while inserting an array?.
Ans. To insert the new array along row-wise we will use axis=0 and add along
column-wise use axis=1.