Hey, welcome back to our new topic Java Variables of java tutorial series, please visit once the previous article of Java Introduction, if you are not familiar with other programming languages then you should know first that what are variables? – Variables are works as a container for storing the values.
Java Variables
Like other programming languages, java also has Variables and data types. Java variables are not different from other programming languages’ variables it’s working the same way but the syntax and the assigning way is different from other programming languages. A Java variable is only a name given to the memory location. In java variables are must be declared before assigning the value in it. It is case sensitive.
In Java, there are three types of Java variables. They are-
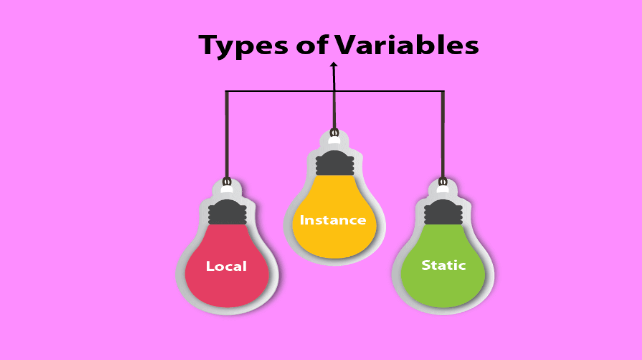
Local Variable in Java:
Local variables are declared inside of the method. These variables are only usable inside the method that declared it. Other methods in the class aren’t even know that the variable exists.
public class main
{
public static void main(String[] args)
{
String myMessage;
myMessage = "Hello, World!";
System.out.println(myMessage);
}
}
We don’t need to specify static on a declaration of a local variable. If we do, the compiler generates an error message and refuses to compile the program.
Instance Variables in java:
This java variable is defined in a class. These are Object-specific variables. It is hold the value which can used by more than one method, block or constructors An instance variable is more alike to class variable. Instance variables belong to an instance of a class. It means instance variables belong to an object and we know that an object is an instance of a class. Every object of a program has it’s own copy of the instance variables. Ignore class and object if you new in programming world we discuss it in the coming tutorial.
When you create a new object of the class you create an instance. Consider, if you have a PEN class, then
class Pen
{
String penName;
int penPrice;
}
Static Variable in java:
It is initialized only once, at the beginning of the program execution. These variables should be initialized first, before the initialization of any instance variables. Static Variables are also called a class variables because they are associated with the class and common for all the instances of class. As for example, If we create three objects of a class and access this static variable, it would be common for all, the changes made to the variable using one of the object would reflect when access it through other objects.
Example of all three variables:
class Main{
static int a = 10; //static variable
int data = 100; //instance variable
void method() {
int b = 20; //local variable
}
}
How to declare a variable in java?
To creating a variable in java, we must specify the type and then assign value in it:
Syntax:
variable type variableName = value;
Example:
public class Main {
public static void main(String[] args) {
String myName = "Hello World";
System.out.println(myName);
}
}
Output:
Hello World
FAQs:
What is class variables?
Java class variables are common to all objects in a class, if any changes are made to these variables via the object, it will reflect in other objects as well.
What is the naming conventions of a Java variables?
1. Java Variables name can’t contain the white spaces, for example: int num ber = 200; is invalid because the variable name has space in it.
2. Java Variables name can start with special characters like $.@, etc.
3. Variable name should start with a lower case letter, for example: int number; For long variables names that has more than one words do it like: int myNumber; int bigNumber; (start the second word with capital letter).
What is JAR file?
A JAR file is a file that contains compressed(Zip) version of .class files, audio files, image files or directories.