Hello friends, welcome back to our new tutorial of pandas Operations in Python tutorial Series. Here we will discuss the Different pandas operations like how to apply functions, getting value of columns and rows, merging, joining and coenacting etc.
Pandas Operations:
There are lots of operations with pandas that will be really useful to you, but don’t fall into any distinct category. Let’s show them here in this lecture:
import pandas as pd
df = pd.DataFrame({'col1':[1,2,3,4],'col2':[444,555,666,444],'col3':['abc','def','ghi','xyz']})
df.head()

How to get information’s on Unique Values?
df['col2'].unique()
Output:
array([444, 555, 666])

Applying Functions
def times2(x):
return x*2
df['col1'].apply(times2)
Output:
0 2
1 4
2 6
3 8
Name: col1, dtype: int64
Permanently Removing a Column from the dataset:
del df['col1'] #it will delete the column1
Sorting and Ordering a DataFrame:
df.sort_values(by='col2') #inplace=False by default

Merging, Joining and Concatenating
There are 3 main ways of combining DataFrames together: Merging, Joining, and Concatenating. In this lecture, we will discuss these 3 methods with examples.
Lets assume we have 3 datasets df1, df2anf df3.
import pandas as pd
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3'],
'C': ['C0', 'C1', 'C2', 'C3'],
'D': ['D0', 'D1', 'D2', 'D3']},
index=[0, 1, 2, 3])
df2 = pd.DataFrame({'A': ['A4', 'A5', 'A6', 'A7'],
'B': ['B4', 'B5', 'B6', 'B7'],
'C': ['C4', 'C5', 'C6', 'C7'],
'D': ['D4', 'D5', 'D6', 'D7']},
index=[4, 5, 6, 7])
df3 = pd.DataFrame({'A': ['A8', 'A9', 'A10', 'A11'],
'B': ['B8', 'B9', 'B10', 'B11'],
'C': ['C8', 'C9', 'C10', 'C11'],
'D': ['D8', 'D9', 'D10', 'D11']},
index=[8, 9, 10, 11])
Concatenation:
Concatenation basically glues together DataFrames. Keep in mind that dimensions should match along the axis you are concatenating on. You can use pd.concat and pass in a list of DataFrames to concatenate together:
pd.concat([df1,df2,df3])

Merging:
We have taking two datasets for this pandas operations. The merge function allows you to merge DataFrames together using similar logic as merging SQL Tables together. For example:
left = pd.DataFrame({'key': ['K0', 'K1', 'K2', 'K3'],
'A': ['A0', 'A1', 'A2', 'A3'],
'B': ['B0', 'B1', 'B2', 'B3']})
right = pd.DataFrame({'key': ['K0', 'K1', 'K2', 'K3'],
'C': ['C0', 'C1', 'C2', 'C3'],
'D': ['D0', 'D1', 'D2', 'D3']})
pd.merge(left,right,how='inner',on='key')
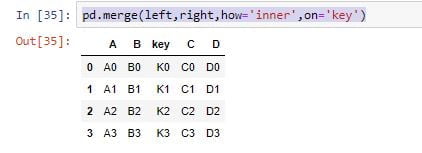
Joining
Joining is a convenient method for combining the columns of two potentially differently-indexed DataFrames into a single result DataFrame.
left.join(right)
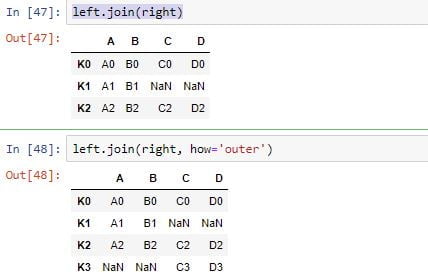
Great Job!
We have touched almost all points of Pandas Operations. If you do have any questions regarding this topic then please contact us through the comment section or you can also mail us.
If you missed the previous lectures on Pandas then please have a look.
Thanks 🙂