Java arrays are not very different from other programming languages; only a little syntax and can vary. In this, arrays in the java article we will learn a small introduction of an array, what is the syntax of java arrays? and we will do some practical examples.
What is a Java array?
Arrays are an object which contains the similar type of data in a contiguous memory location. It is nothing but a data structure where we store similar elements. We can store only a fixed set of values in an array. Java arrays are index-based the first element’s position is stored at the 0th index, 2nd the one is stored on the 1st index and respectively. This function is different from c and c++.
Java array indexing example
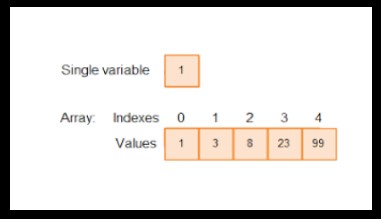
Syntax:
We can declare a array in two ways:
1) data type [size] variable;
Variable=new datatype[size];
2) data type var-name[];
variable = new datatype[];
Types of Array in java
In java, Two types of arrays are present, they are:
- Single dimensional array
- Multi-dimensional array
Single dimensional array
In the above snapshot, we have shown the indexing of a single dimension array.
Integer array Example:
public class ArrayExample {
public static void main(String[] args) {
int value = 7;
int[] values;
values = new int[3];
System.out.println(values[0]);
values[0] = 1;
values[1] = 2;
values[2] = 3;
System.out.println(values[0]);
System.out.println(values[1]);
System.out.println(values[2]);
for(int i=0; i < values.length; i++) {
System.out.println(values[i]);
}
int[] numbers = {5, 6, 7};
for(int i=0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
}
}
Output:
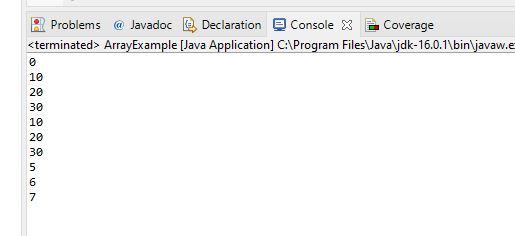
String array Example:
public class ArrayExample {
public static void main(String[] args) {
// Declare array of (references to) strings.
String[] words = new String[3];
// Set the array elements (point the references
// at strings)
words[0] = "Hello";
words[1] = "to";
words[2] = "you";
// Access an array element and print it.
System.out.println(words[2]);
// Simultaneously declare and initialize an array of strings
String[] fruits = {"apple", "banana", "pear", "kiwi"};
// Iterate through an array
for(String fruit: fruits) {
System.out.println(fruit);
}
}
}
Output:
you
apple
banana
pear
kiwi
Multi-Dimensional Arrays:
When we want to print a matrix or use rows and columns, we can use a multi-dimensional array. Simply we can that multi-dimensional array – data is stored in a row and column-based indexes.
public class ArrayExample {
public static void main(String[] args) {
// 1D array
int[] values = {3, 5, 2343};
// Only need 1 index to access values.
System.out.println(values[2]);
// 2D array (grid or table)
int[][] grid = {
{3, 5, 2343},
{2, 4},
{1, 2, 3, 4}
};
// Need 2 indices to access values
System.out.println(grid[1][1]);
System.out.println(grid[0][2]);
// Can also create without initializing.
String[][] texts = new String[2][3];
texts[0][1] = "Hello there";
System.out.println(texts[0][1]);
// How to iterate through 2D arrays.
// first iterate through rows, then for each row
// go through the columns.
for(int row=0; row < grid.length; row++) {
for(int col=0; col < grid[row].length; col++) {
System.out.print(grid[row][col] + "\t");
}
System.out.println();
}
// The last array index is optional.
String[][] words = new String[2][];
// Each sub-array is null.
System.out.println(words[0]);
// We can create the subarrays 'manually'.
words[0] = new String[3];
// Can set a values in the sub-array we
// just created.
words[0][1] = "hi there";
System.out.println(words[0][1]);
}
}
Output:
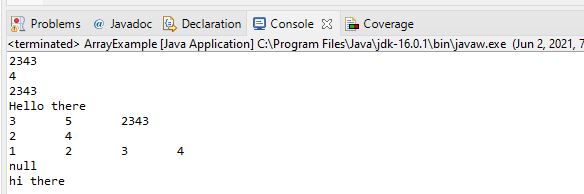
All these examples are the examples of static array where we initialized the size of the array. After this we are showing the example of dynamic array.
import java.util.Scanner;
public class ArrayExample {
public static void main(String[] args) {
int i,size ;
Scanner scanner= new Scanner(System.in);
System.out.println("Enter the size of array: ");
size = scanner.nextInt();
int a[]=new int[10];
for(i=0; i<size; i++) {
a[i]= scanner.nextInt();
}
System.out.print("Print array");
for( i=0; i<size; i++) {
System.out.println(a[i]);
}
}
}
Output:
0
1
2
3
4
5
6
7
8
9
Java ArrayList:
Arraylist is nothing but a class which uses a dynamic array to store the value. It has no size limit we can add remove the element anytime. It is present in the java.util package. It might be slower than a normal array but it can be helpful in programs where lots of manipulation in the array is needed.
ArrayList class declaration
import java.util.ArrayList; // importing the ArrayList class
ArrayList<String> cars = new ArrayList<String>(); //Creating an ArrayList object called cars that is storing strings
This is small introduction of arraylist we will discuss it in separate post.
FAQs:
1. What is the difference between static and dynamic arrays?
1. In a static array the size of the array is fixed while a dynamic array doesn’t have a fixed size.
2. Static Arrays are declared before runtime and are also assigned values while writing the code.
2. Can we pass a negative value in Array size?
No, it is not possible. If we pass a negative value in Array size then we will get the NegativeArraySizeException at the run time.
3. Where does Array stored in the JVM memory ?
As we know array is a object. So, it is stored in heap memory in the JVM.
4. What is ArrayStoreException ? When this exception is occurred?
ArrayStoreException is a runtime exception. The array must contain the same data type elements.
This exception is thrown to indicate that an attempt has been made to store the wrong type of object into an array of objects. In other words, if you want to store the integer Object in an Array of String you will get the ArrayStoreException.
Source: Oracle docs,