In this article you will the different operators in java, their syntax and how to use them with the help of some examples.
What are Operators in java?
Operators in Java are a symbol that is used to perform operations on values and variables. For example: +, -, *, / etc. Like other programming languages java also rich in operators.
There are many kind of operators in Java:
- Arithmetic Operators
- Assignment Operators
- Relational Operators
- Logical Operators
- Unary Operators
- Bitwise Operators
Arithmetic operators:
Arithmetic operators in java are used to perform arithmetic operations on variables and values. For instance:
a + b;
Here the list of some Arithmetic operators:
+ | Addition |
- | Subtraction |
* | Multiplication |
/ | Division |
% | Modulo Operation (Remainder after division) |
Example of Java Arithmetic Operators:
public class Main {
public static void main(String[] args) {
// declare variables
int x = 22, y = 15;
// addition operator
System.out.println("x + y = " + (x + y));
// subtraction operator
System.out.println("x - y = " + (x - y));
// multiplication operator
System.out.println("x * y = " + (x * y));
// division operator
System.out.println("x / y = " + (x /y));
// modulo operator
System.out.println("x % y= " + (x % y));
}
}
Output:
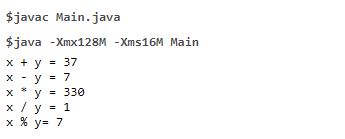
Assignment Operators in java:
Java assignment operator is one of the most common operators. It is used to specify the value on its right to the operand on its left. For example:
class OperatorExample{
public static void main(String args[]){
int a=10;
int b=10;
//a+=b;//a=a+b internally so fine
a=a+b;//Compile time error because 10+10=20 now int
System.out.println(a);
}}
Output will be: 20
Here some of the assignment operators:
Operators Example Example Explanation
= | a = b; | a = b; |
+= | a += b; | a = a + b; |
-= | a -= b; | a = a - b; |
*= | a *= b; | a = a * b; |
/= | a /= b; | a = a / b; |
%= | a %= b; | a = a % b; |
Relational Operators in java:
Java Relational operators are not unlike from other programming languages. Relational operators are basically used for show the relations between the values. Just for example: less than(<) and greater than sign(>).
List of some relational operators in java:
Operator | Description | Example |
---|---|---|
== | Is Equal To | 2 == 5 returns false |
!= | Not Equal To | 2 != 5 returns true |
> | Greater Than | 2 > 5 returns false |
< | Less Than | 2 < 5 returns true |
>= | Greater Than or Equal To | 2 >= 5 returns false |
<= | Less Than or Equal To | 2 <= 5 returns false |
Logical Operators
Java Logical operators are used in decision making operations, to check weather the value or expression is true or false.
Operator | Example | Meaning |
---|---|---|
&& (Logical AND) | expression1 && expression2 | true only if both expression1 and expression2 are true |
|| (Logical OR) | expression1 || expression2 | true if either expression1 or expression2 is true |
! (Logical NOT) | !expression | true if expression is false and conversely |
Example:
class OperatorExample{
public static void main(String args[]){
// && operator
System.out.println((5 > 3) && (8 > 5)); // true
System.out.println((5 > 3) && (6 < 5)); // false
// || operator
System.out.println((5 < 3) || (8 > 5)); // true
System.out.println((5 > 3) || (7 < 5)); // true
System.out.println((5 < 3) || (8 < 5)); // false
// ! operator
System.out.println(!(6 == 3)); // true
System.out.println(!(5 > 3)); // false
}
}
Unary Operators
The Java unary operators works with only one operand. Unary operators are used to perform several operations i.e.:
- incrementing/decrementing a value by 1
- negating an expression
- inverting the value of a boolean
Example:
class OperatorExample{
public static void main(String args[]){
int a=10;
System.out.println(a++);//10 (11)
System.out.println(++a);//12
System.out.println(a--);//12 (11)
System.out.println(--a);//10
}}
Output:
10
12
12
10
Bitwise Operators
Java Bitwise operators are used to perform operations on individual bits. Just For example,
Bitwise complement Operation of 35
35 = 00100011 (In Binary)
~ 00100011
________
11011100 = 220 (In decimal)
List of the Bitwise Operators in Java:
Operator | Description |
---|---|
| | Bitwise OR |
& | Bitwise AND |
^ | Bitwise XOR |
~ | Bitwise Complement |
<< | Left Shift |
>> | Signed Right Shift |
>>> | Unsigned Right Shift |
This is all about Operators in java, in the next article we will see more topics on java tutorials. If you are not visited our previous articles of this java tutorial series then click here.
Q. Why we use curly braces every time in java code?
Ans: Every time we use curly braces in java to create different blocks or regions.
Q. What is Compound Assignment Operator?
Ans: The operator that combines Arithmetic operator with assignment is called Compound Assignment operator. Example: a+=10;