Like other programming languages, java also have three types loops which we will going to see in this Loops in java tutorials. Lets get Started …..
What are Loops in Java?
The main work of loops is to execute a set of instructions repeatedly when some conditions become true or false. Java Loops are three types:
- For Loop
- Do… while Loop
- While Loop
Java For Loop
Java for loops is used to iterate a part of the program several times. If we know the number of iteration is fixed, it is recommended to use for loop.
For loop syntax in Java:
for(initialExpression; testExpression; incr/decr){
//code to be executed
}
Java for loop example:
// print sequencely 10 numbers
public class Forloopexample{
public static void main(String[] args){
int i;
for (i=0; i<10; i++){
System.out.println(i);
}
}
}
Java While Loop:
The While loops in java are used to iterate a part of the program several times. If the number of iteration is not fixed, it is good to use a while loop.
Java While Loop Syntax:
while(loop condition){
//code to be executed
}
Java while Loop Example:
public class WhileloopExample {
public static void main(String[] args) {
int i=1;
while(i<=10){
System.out.println(i);
i++;
}
}
}
Output:
1
2
3
4
5
6
7
8
9
10
Java do-while Loop
A java do while loop is similar to while loop except that a do…while loop is guaranteed to execute at least one time.
Java do-while Loop syntax:
do{
//code to be executed
}while(condition);
Java do-while Loop example:
public class DoWhileExample {
public static void main(String[] args) {
int i=1;
do{
System.out.println(i);
i++;
}while(i<=10);
}
}
Output:
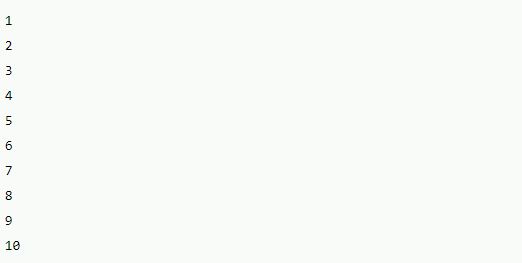
1. What is infinity loop in java?
An infinite loop in java is a piece of coding that does not have a functional exit so that it repeats endlessly. For example, if we want to make for infinity loop then we have to use two semicolons (;; )in the for loop, it will be infinitive for the loop.
Syntax:
for(;;){
//code
}
2. When we should use for and Do-while loop?
When we know the no. of iteration then for loop is better to use. And when we don’t know the no. of iteration then we can use Do-while loop.
This is all about the loops in java. In this article, we have discussed the java for loop, Do-while loop, and while loop. If you missed the previous article on java control statements.