In this article, we will know about what is inheritance in java? types of inheritance, use of inheritance with examples.
What is Inheritance in Java?
Inheritance is a concept that gets all the properties and behavior from parent class to other child classes; As for example relationship between Son and his Father. This is an important property of Oops concept of java.
In Java Inheritance, a class can inherit properties and methods from another class. The class that acquires the properties is known as the sub-class or the child class. The class from which the properties are inherited is known as the parent class or superclass.
Inheritance in java represents the IS-A relationship which is also known as a parent-child relationship.
Syntax:
class child-class extends parent-class
{
//methods
//fields
}
Through extends keywords, we can inherit classes. The extends indicates that making a new class that derives from an existing class.
Program example:
class InheritanceExample {
int var1=1000;
}
class Inherite extends InheritanceExample{
int var2 =2000;
public static void main(String[] args) {
Inherite e= new Inherite();
System.out.println(e.var1);
System.out.println(e.var2);
}
}
Output:
1000
2000
Why use Inheritance?
The main advantage is code reusability and also method overriding (runtime polymorphism).
Type of Java Inheritance:
There are 3 types of inheritance present in java, they are:
- Single Inheritance
- Multiple Inheritance
- Multi-Level Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
Single Inheritance in java
Creating child classes from a single parent class are called single inheritance.

class Animal{
void eat(){
System.out.println("Dog is eating");
}
}
class Dog extends Animal{
void bark(){
System.out.println("Dog is barking");
}
}
class TestAnimal{
public static void main(String args[]){
Dog d=new Dog();
d.bark();
d.eat();
}}
Output:
Dog is eating
Dog is barking
Multi-level Inheritance
When a class extends the main class, which extends another class then this process of extension is called multilevel inheritance. For example, if one class Z extends class Y and class Y extends class X, then this type of inheritance is multilevel inheritance.

It’s pretty clear as we can see in the diagram that Multilevel is a concept of grandparent class.
Example:
class Car{ public Car() { System.out.println("The Car"); } public void vehicleType() { System.out.println("Vehicle Type is: Car"); } } class Maruti extends Car{ public Maruti() { System.out.println("The Maruti"); } public void brand() { System.out.println("Car Brand is: Maruti"); } public void speed() { System.out.println("Max Speed is: 90Kmph"); } } public class Maruti800 extends Maruti{ public Maruti800() { System.out.println("Maruti Model is: 800"); } public void speed() { System.out.println("Max Speed is: 80Kmph"); } public static void main(String args[]) { Maruti800 obj=new Maruti800(); obj.vehicleType(); obj.brand(); obj.speed(); } }
Output:
The Car
The Maruti
Maruti Model is: 800
Vehicle Type is: Car
The Brand: Maruti
Max Speed is: 80Kmph
Hierarchical Inheritance
When two or more classes inherit a single class, it is known as hierarchical inheritance.
class Animal{
void eat(){System.out.println("eating");}
}
class Dog extends Animal{
void bark(){System.out.println("barking");}
}
class Cat extends Animal{
void meow(){System.out.println("meowing");}
}
class TestInheritance3{
public static void main(String args[]){
Cat c=new Cat();
c.meow();
c.eat();
}}
Output:
meowing
eating
Multiple Inheritance
The “Multiple Inheritance” refers to the concept of one class inherits (Or extends) more than one base class.
Class X
{
public void methodX()
{
System.out.println("Class X method");
}
}
Class Y extends X
{
public void methodY()
{
System.out.println("class Y method");
}
}
Class Z extends Y
{
public void methodZ()
{
System.out.println("class Z method");
}
public static void main(String args[])
{
Z obj = new Z();
obj.methodX(); //calling grand parent class method
obj.methodY(); //calling parent class method
obj.methodZ(); //calling local method
}
}
Output:
Class X method
Class Y method
Class Z method
Hierarchical Inheritance
In Inheritance in Java, more than one derived class extends a single base class. In simple words, more than one child class extends a single parent class or a single parent class has more than one child class.
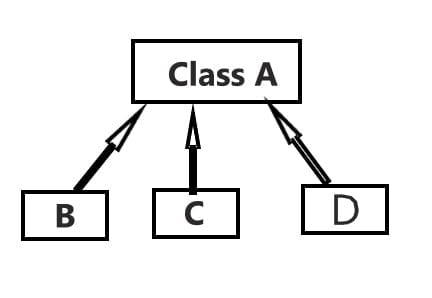
class A
{
public void methodA()
{
System.out.println("The method of Class A");
}
}
class B extends A
{
public void methodB()
{
System.out.println("The method of Class B");
}
}
class C extends A
{
public void methodC()
{
System.out.println("The method of Class C");
}
}
class D extends A
{
public void methodD()
{
System.out.println("The method of Class D");
}
}
class JavaExample
{
public static void main(String args[])
{
B obj1 = new B();
C obj2 = new C();
D obj3 = new D();
obj1.methodA();
obj2.methodA();
obj3.methodA();
}
}
Output:
method of Class A
method of Class A
method of Class A
Why Multiple Inheritance is not supported in Java?
Java does not support multiple inheritances because of two reasons:
- In java, every class is a child of
Object
class. When it inherits from more than one super class, sub class gets the ambiguity to acquire the property of Object class.. - In java every class has a constructor, if we write it explicitly or not at all. The first statement is calling
super()
to invoke the supper class constructor. If the class has more than one super class, it gets confused.
Source: StackOverflow