Hello everyone and welcome to the Numpy Array operations lecture and this lecture we’re going to show you basic operations reform on umpire arrays.
Python Numpy Array Operations
Like a normal Python List array, a NumPy array has also various operations like arithmetic operations.
Let’s go ahead and jump to the Jupiter notebook to get start the Arithmetic operations on Numpy Array Operations.
Arithmetic
We can easily perform array with array arithmetic, or scalar with array arithmetic. Let’s see some examples:
import numpy as np
arr = np.arange(0,10)
#adding array with itself
arr + arr
Output: array([ 0, 2, 4, 6, 8, 10, 12, 14, 16, 18])
#multiplication of array
arr*arr
Output:
array([ 0, 1, 4, 9, 16, 25, 36, 49, 64, 81])
# substraction
arr -arr
Output:
array([0, 0, 0, 0, 0, 0, 0, 0, 0, 0])
# exponential
arr**3
Output: array([ 0, 1, 8, 27, 64, 125, 216, 343, 512, 729])
Here, something I need to say if we divide the array by itself. The output will come with a warning that is not an error, This also applicable when we divide an array with infinity. Below given snapshot shows how it works:
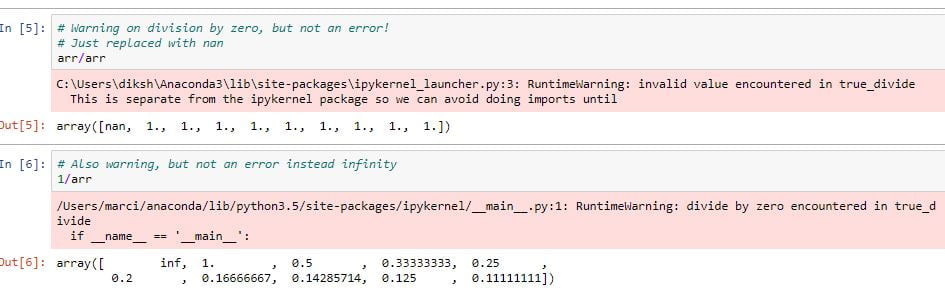
Universal Array Functions
Numpy comes with many universal array functions, which are essentially just mathematical operations you can use to perform the operation across the array. Let’s show some common ones:
#Taking Square Roots
np.sqrt(arr)
Output:
array([ 0. , 1. , 1.41421356, 1.73205081, 2. ,
2.23606798, 2.44948974, 2.64575131, 2.82842712, 3. ])
#Calcualting exponential (e^)
np.exp(arr)
Output:
array([ 1.00000000e+00, 2.71828183e+00, 7.38905610e+00,
2.00855369e+01, 5.45981500e+01, 1.48413159e+02,
4.03428793e+02, 1.09663316e+03, 2.98095799e+03,
8.10308393e+03])
np.max(arr) #same as arr.max()
Output: 9
# for find log
np.log(arr)
Output:
array([ -inf, 0. , 0.69314718, 1.09861229, 1.38629436,
1.60943791, 1.79175947, 1.94591015, 2.07944154, 2.19722458])
Note: it’s also contain warning message.

This is all about the NumPy array operations with some code examples. I hope you like it. Don’t forget to read the previous articles on the NumPy tutorial.
FAQS:
Is a list mutable or immutable and can its values be changed?.
Ans. List is mutable, we can change the value.
What is a Python Set?
Ans. Set is nothing but a collection that is unordered and unindexed. And it returns no duplicate members. So when you print the values of the Set, you won’t get it in the same order as you have created.
Can we remove more than one object from a Python list?
Ans.Yes! We can remove multiple elements. In best practice write a loop and remove the elements.