Welcome, everyone, to this section of the course on advanced python modules and we are going to start with Python Collection Module.
Python has several built-in modules that we haven’t really fully explored yet. In this section, we will dive deeper into some useful built-in modules that already come prepackaged with Python.
We’ll explore their use cases.
The modules will be covered in the section, the course is:
- The collections module,
- The OS module
- Math and Random and the Datetime module.
- Python debugger
- Timeit and in general, how to time your code.
- Regular expressions and how to search for patterns in text.
- How to unzip and zip files with Python through built-in modules and also modules
Python Collection Module:
Based on Python, and it implements specialized container data types that are essentially alternatives to Python built-in containers that are just general purpose. So what I mean by a container is something like a dictionary or a tuple. The container is nothing but an object that uses for storing the different objects and provides a way to access the contained objects.
We’ve already learned how to use normal dictionaries. But Counter has specialized dictionary objects. So we’re going to learn about these specialized container types.
You may not always need these specialized versions, but in certain use cases, you may find them useful. Let’s go ahead and open up the Jupiter notebook.
The first object I want to show you from the collections module is the counter class.
Counter Class:
We will import it by saying from collections import counter.

Let’s imagine you have a situation where you have a list and then some unique values in the list. But there’s also repeats for these unique values.
So I’m just gonna make a bunch of A, B, and C and so on. Now, I wanted you to get a count of each unique item in this list.
For example, I want you to count how many A are there? How many B are there? How many C are there?
Well, you currently do have the skills to do this with counter class.
Notice: this looks very similar to a dictionary and counters technically a dictionary subclass that essentially just helps count cashable objects. So inside of it, elements are stored as dictionary keys and the counts of the objects are stored as the values.
Example.2: let’s create a sentence here saying ” how many times does each word shows up in this sentence with aword or something like that?”
Well, I know I can’t simply split this string on the white space to get a list that looks like this, which means I can then call counter on that list. And I have a count of each word I see here. Words showed up twice.
Code:
sentence = "how many times does each word shows up in this sentence with aword or something like that?"
Counter(sentence.split())
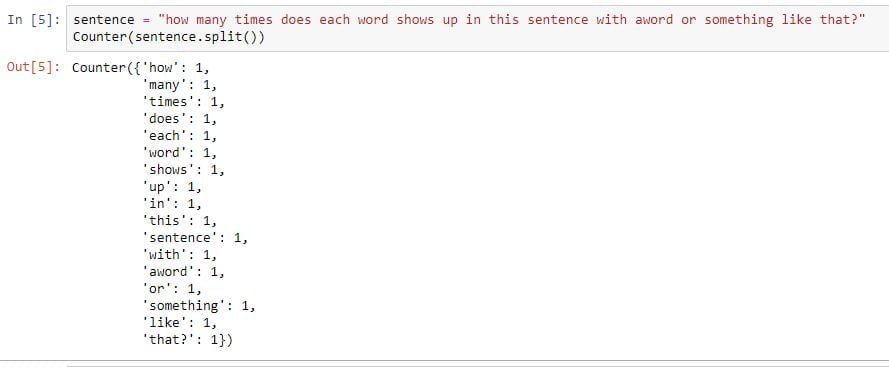
Well, We have two more things to show you from the collections module.
The second one is the default dictionary(defaultdict).
Default dictionary(defaultdict)
A DefaultDict is also a sub-class to the dictionary. the main use of it to provide some default values for the key which does not exist and never raises a KeyError.
Let me show you how we can create this default dictionary.
from collections import defaultdict
# Defining a defaultdict
d = defaultdict(list)
for i in range(6):
d[i].append(i)
print("The values as list:")
print(d)
Output:

NamedTuple
A NamedTuple returns a tuple object with names for each position that the ordinary tuples not able to do.
The named tuple tries to expand on a normal tuple object by actually having named indices. So let me show you what I mean by that.
Example:
from collections import namedtuple
Dog= namedtuple('Dog', ['name', 'age', 'bread'])
sam= Dog('Sam', '5', 'Huskey')
print(sam)
Output:

The last specialized container object that I want to show you from the python collection module is called a ChainMap in Python.
ChainMap in Python
Python contains a container that is called “ChainMap” which encapsulates many dictionaries into one (unit). ChainMap is a member of the Python collections module. For instance:
from collections import ChainMap
d1 = {'a': 1, 'b': 2}
d2 = {'c': 3, 'd': 4}
d3 = {'e': 5, 'f': 6}
# Defining the chainmap
c = ChainMap(d1, d2, d3)
print(c)
Output:

Must Read:
All right. So that’s it for this topic, we have discussed the Python collection module with suitable examples. I hope these can really help you and I will see you at the next topic.
Thank you 🙂