In this article, we are going to discuss Python Exception Handling. In the previous one, we had discussed the oops concept, inheritance, and polymorphism.
What is Python Exception?
An exception
is an error that occurs during the execution of a program. Errors are two types of syntax error ( it is occurred due to the wrong syntax of language) and exception error ( it has occurred when the program is syntactically correct but after the code resulted in an error. ).
Python allows a programmer to handle such exceptions using try … except clauses, thus avoiding the program to crash.
Some of the python expressions, though written correctly in syntax, result in errors during execution. Such scenarios have to be handled.
Sample Python Exceptions
- Try executing the following two expressions 10 + ( 1 / 0)36 + ’20’
- The first one throws an error message,
ZeroDivisionError : division by zero
, and - Second one throws,
TypeError : unsupported operand type(s) for +: 'int' and 'str'
.

In Python, every error message has two parts. The first part tells what type of exception it is and the second part explains the details of the error.
Python Exception Handling
- Python handles exceptions using code written inside
try ... except
blocks. - A
try
block is followed by one or moreexcept
clauses. - The code to be handled is written inside
try
clause and the code to be executed when an exception occurs is written insideexcept
clause.
Sample Exception Handling
Example 1:
try:
a = pow(2, 4)
print("Value of 'a' :", a)
b = pow(2, 'hello') # results in exception
print("Value of 'b' :", b)
except TypeError as e:
print('oops!!!')
print('Out of try ... except.')
Output

- You can observe from the output that execution of statements, present, after exception are skipped.
Raising Exceptions
The raise
keyword is used when a programmer wants a specific exception to occur.
Example 2:
try:
a = 2; b = 'hello'
if not (isinstance(a, int)
and isinstance(b, int)):
raise TypeError('Two inputs must be integers.')
c = a**b
except TypeError as e:
print(e)
Output
Two inputs must be integers.
- Above example raises
TypeError
when botha
orb
are not integers.
User Defined Functions
- There are many built-in exceptions in Python, which are directly or indirectly derived from
Exception
class. - Python also allows a programmer to create custom exceptions, derived from base
Exception
class.
Example 1:
class CustomError(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return str(self.value)
try:
a = 2; b = 'hello'
if not (isinstance(a, int)
and isinstance(b, int)):
raise CustomError('Two inputs must be integers.')
c = a**b
except CustomError as e:
print(e)
Output
Two inputs must be integers.
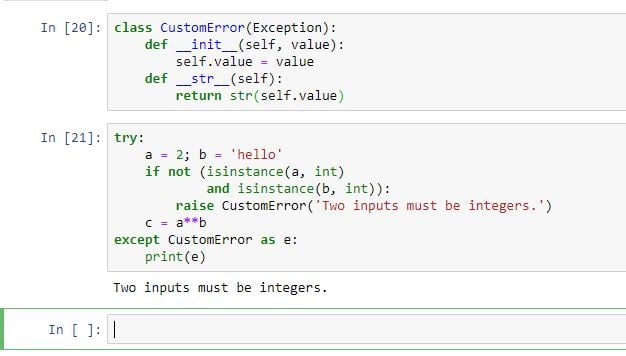
CustomError
is raised in above example, instead ofTypeError
.
Using ‘finally’ clause
The finally
clause is an optional one that can be used with try ... except
clauses.
All the statements under finally
clause are executed irrespective of exception occurrence.
Example 1
def divide(a,b):
try:
result = a / b
return result
except ZeroDivisionError:
print("Dividing by Zero.")
finally:
print("In finally clause.")
print('First call')
print(divide(14, 7))
print('Second call')
print(divide(14, 0))
Output
First call
In finally clause.
2.0
Second call
Dividing by Zero.
In finally clause.
None
- Statements inside
finally
clause are executed in both function calls.
Using ‘else’ clause
else
clause is also an optional clause withtry ... except
clauses.- Statements under
else
clause are executed only when no exception occurs intry
clause.
Example 2
try:
a = 14 / 7
except ZeroDivisionError:
print('oops!!!')
else:
print('First ELSE')
try:
a = 14 / 0
except ZeroDivisionError:
print('oops!!!')
else:
print('Second ELSE')
Output
First ELSE
oops!!!
FAQs on Python Exception Handling:
How many except statements can try-except block we have?
More than zero but we have to take at least one in the block.
Is the below following Python code valid or not?
try:
#code
except:
# code
else:
# code
Yes, it is valid.
What happens when ‘1’ == 1 is executed?
We get an error:
‘1’ == 1
^ SyntaxError: invalid character in identifier
What will be the output of the following Python code?
x=10
y=20
assert x>y, ‘X too small’
You will get a error: AssertionError: X too small
In this article we have successful executed the python exception handling with their type with suitable examples. In the coming one we will discuss about the Python modules.
Thank You 🙂