Hey!! Friends welcome to our new article on the Javascript tutorial. Here we will start from where we finished in the previous article. In the previews article, we talk about what is javascript, History of its, etc.
Here we started the tutorial with Syntax , console and datatypes.
If you missed the previous articles, then check once Introduction to Javascript.
What’s inside: Developer Environment Console Syntax JavaScript Data Types FAQs: |
Developer Environment
What do you require to develop JavaScript? The explanation is simple – any web browser such as Internet Explorer, Chrome, or Firefox.
Most browsers come up with developer tools that allow us to,
- View the page that is rendered on the browser
- View the HTML and JavaScript code
- Debug JavaScript code
- View the console tab to take input commands.
Console
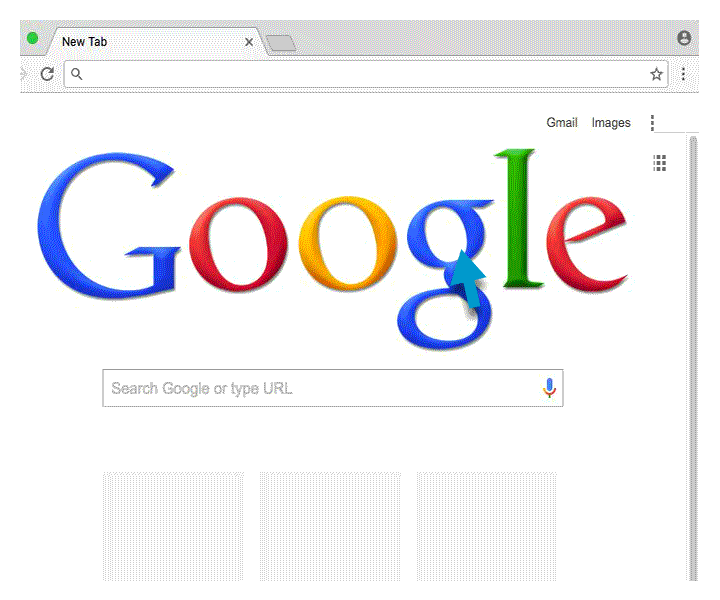
The console is a simple text interface to input commands and view output. You can reach the console by operating to the Developer Tools menu from the browser options. This comes usable for debugging.
Simply say console.log(“Hello World”);
and try it out.
Syntax
- JavaScript is an interpreted language and needs no compilation.
- JavaScript is case sensitive and develops statements. It means that the language keywords, variables, function names, and any other identifiers must always be typed with a consistent capitalization of letters.
- The semicolon is the most reliable way to group the reports.
- JavaScript is insensitive to whitespace.
- Script tags, including JavaScript code, are best located at the end of the code.
- Comments start with // or /* and */
The script tag takes two essential properties −
- Language − This attribute defines what scripting language you are using. Typically, its content will be javascript. Although recent versions of HTML (and XHTML, its successor)have phased out this attribute’s use.
- Type − This attribute uses for recommended to indicate the scripting language in use, and its value should be set to “text/javascript.”
So, it is look like:
<script language = "javascript" type = "text/javascript">
JavaScript Code
</script>
JavaScript Data Types

In programming, data models are a primary concept. For operating variables, it is essential to know about what is the type of variable.
Without data types, a computer cannot safely solve this:
var x = 16 + "blog";
Does it make any sense to add “blog” to sixteen? Will it produce an error, or will it give output?
JavaScript will treat this as:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript</h2>
<p>When we are adding a number and a string, JavaScript will treat the number as a string.</p>
<p id="demo"></p>
<script>
var x = 16 + "blog";
document.getElementById("demo").innerHTML = x;
</script>
</body>
</html>
JavaScript
When we are adding a number and a string, JavaScript will treat the number as a string.
Primitive JavaScript data types:
- String [ “Howdy”]
- · Number [ 23, 482.038]
- · Boolean [ true, false ]
- · Null [ null value ]
- · Undefined [ value not defined ]
- · Objects
Number, String, Boolean
- Numbers are only double-precision 64-bit values. There are no integers in JS. For example – 42 or 42.39794
- · Strings are to store characters.
- · Booleans are true and false.
Null, Undefined
- Undefined is different from JavaScript. It is a data type allowed to variables that are declared as variables but not assigned any values.
- Null is a sign to denote the null value.
JavaScript Objects
The objects of JavaScript are written with curly braces {}. Object properties are written as like name: value pairs, separated by commas.
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Objects</h2>
<p id="example"></p>
<script>
var person = {
firstName : "lucine",
lastName : "James",
age : 25,
eyeColor : "brown"
};
document.getElementById("example").innerHTML =
person.firstName + " is " + person.age + " years old.";
</script>
</body>
</html>
Output of the following code:
JavaScript Objects
FAQs: in Javascript Tutorial
1. What option would you specify when inserting the code script type=”____” on a web page?
Ans: text/JavaScript
2.______________ is used to display whether a value is a number or not.
Ans: isNaN()
3. Syntax of alert?
Ans: alert(“Hello! I am Lucine!!”);
All the mentioned topics are the basics parts of the javascript tutorial. In the forthcoming article, we will cover variables, Operators, and so on. Please stay with us on this journey :).
Your post was really helpful. Javascript is explained very well by you 👍
Thanks, For more please stay with us 🙂
Valuable information…thnx for sharing
Nice article
Helpful tips
Informative always keep it up good work ❤️
Quite informative