After Python Loops, now its turn of Python Functions. So guys, in this article, we will discuss what Function is in Python? How it is working with suitable examples.
Let’s start the tutorial…
Table of Content: Python Functions Introduction How to create a function? Calling a Function Iterators List Comprehensions in Python Generators FAQsLoop |
Python Functions Introduction:
Function in Python is comparable to other programming languages. A function is a block of code that will only run when it is called.
Python Functions are two types:
- User defined Functions
- Built in Functions
As we already know, Python gives many built-in functions like print() function, etc., but we can also create our functions. Functions are created by the user, which are called user-defined functions. The Function contains the set of programming statements enclosed by {}.
How to create a function?
We can define a function in Python by using the def keyword.
Syntax:
def function_name (parameters):
function_code block
return <value>
Syntax explanation:
- The def keyword is applied to define the Function.
- function_name is the function name that will anything Function name is up to the user.
- A function accepts the parameter, and it can be optional.
- The function block is started with the colon (:).
- The return statement is used to return the value.
Example.1:
def lesser_of_two_number(a,b):
if a%2==0 and b%2==0:
if a<b:
result=a
else:
result=b
else:
if(a>b):
result=b
else:
result=a
return result

Example.2:
def animal_cracker(text):
wordlist=text.split()
#s=wordlist[0]
#d=wordlist[1]
return wordlist[0][0]== wordlist[1][0]
Output:

Calling a Function
Once we finalized the basic structure of a function, then we can call a function by typing the function name, followed by ().
Syntax:
Function_name()
Example:
def first_function():
print("Hello world")
first_function()
Iterators
- A Python Iterator is an object that allows the programmer to traverse through all the collection elements, regardless of its specific implementation.
- The values of an Iterator can be accessed only once and in sequential order. An example of An iterator is given below:
x = [7, 3, 1]
s = iter(x)
print(next(s)) # -> 7
print(next(s)) # -> 3
print(next(s)) # -> 1
print(next(s)) # -> StopIteration Error
Output:
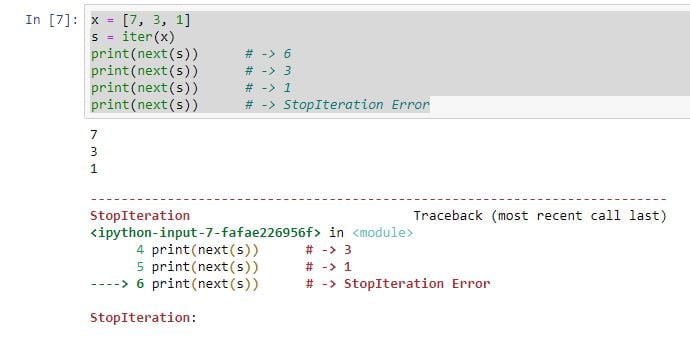
You can see the iteration; in the 6th line, we can see the stopIteration error when the list has no item left.
List Comprehensions
- List comprehensive is an Alternative to for loops.
- More short, readable, efficient, and mimic functional programming style.
- Used to:
- Apply a method to all or specific elements of a list, and
- Filter elements of the list satisfying specific criteria.
Example:
x = [7, 3, 1] y = [ i**2 for i in x ] # List Comprehension expression print(y)
Output will be like this: [49, 9, 1]
Generators in Python
- A Generator object is an iterator whose values are created at the time of accessing them.
- It can be obtained either from a generator expression or a generator function.
Example:
x = [7, 3, 1]
g = (i**2 for i in x) # generator expression
print(next(g))
Output: 49
FAQs:
The output of the expression { ord(i) for i in ‘apple’ } is ____________.
ANS: {97, 112, 108, 101}
The Generators consume more space in memory than the lists. True or False
ANS: False
The elements of an iterator can be accessed multiple times. True or False
ANS: False
What is the output of the below mentioned code?
class A:
x = 0
def __init__(self, a, b):
ANS: Results in Error
Previous Post
We have discussed the Python functions, iterators, list comprehension, and Generators with appropriate examples in the above. In the coming one, we will discuss the lambda and python class and many more.
Thank You 🙂