Hey!! Friends, welcome to our new article of javascript tutorial beginners. Here we will start from where we finished in the previous article. Previously, we talked about If…Else and Loops of javascript Tutorials for Beginners.
Here we started the tutorial with Objects, Date objects and Arrays.
If you missed the previous article, then check once Javascript Tutorial 103.
What’s inside: Objects JavaScript Arrays JavaScript Date Objects FAQs: |
Javascript Objects

- The object in JavaScript is an entity with features and type. A property is a variable connected to the object. These features define the properties of the object.
- For example, a car can be an object with properties colour, model, etc.
- You can access the object’s property using the dot operator.
- In this example, a car is an object with a model as a property.
For example
var car = new Object();
car.model = 'Ford';
console.log(car.model);
Object Properties
The name:values pairs in JavaScript objects are called properties:
objectName.propertyName
For example:
Var obj=new object();
Obj.Firstname:”Jo’’;
Console.log(obj.firstname );
Create an Object in JavaScript:
Create an empty object and then add properties to the object:
For example:
var obj={};
obj.prop1="Hello";
obj.prop2="World";
console.log(obj.prop1 + ' ' + obj.prop2);
By using Object Literal ie. Name value pair:
For example:
var student = {Name:"Ben", age:20};
console.log(student);
- By using keyword
new
Example:
var obj= new Object();
obj.prop1="Hello";
obj.prop2="World";
console.log(obj.prop1 + ' ' + obj.prop2);
Nested Objects
- We can also must an object nested inside different objects, i.e., an object will have another object as its attribute, which has separate properties. Just same the normal objects, these inner objects can also be accessed by applying the dot operator.
Example:
var student = { Name:"Ben", age:20 , degree: {class:"B.Tech",section:"A"}};
We can access the inner object, class
by
console.log(student.degree.class);
Javascript Object Methods
Objects can also have methods.
Methods of JavaScript are actions that can be performed on objects. It is stored in properties as function definitions.
objectName.methodName()
JavaScript Arrays
In the java, script Arrays are used to store a set of values in a single variable. Just like any other language, JavaScript arrays are also represented using square brackets. An array is a special kind of variable. It can hold more than one value at a time.

For example:
var myArray=["Hello", "World"];
And of course, they are accessed using the index, which always starts with 0.
var myString=myArray[0]
In case you access an index for which no value has been assigned, then the console will return undefined. In the previous example, on myArray, which carries only two values, Hello and World, they are assigned to myArray[0] and myArray[1]. Hence, the console will return undefined if you look for myArray[2].
Arrays are predefined objects, and its index is the object properties. Since the index is an integer, we access the object properties using a square bracket instead of a dot operator.
Food for Thought
Following is the code to alert the max number in an array. Can you think of a way to get the result without using loops? Explore yourself…
var numbers = [3,342,23,22,124];
var max = 0;
for(var i=0;i<numbers.length;i++){
if(numbers[i] > max){
max = numbers[I];
}
}
alert(max);
Array Methods
A lot of looping and conditions can be avoided using math and string functions. Please visit JavaScript documentation to learn about these objects
var numbers = [3,342,23,22,124];
numbers.sort(function(a,b){return b - a});
alert(numbers[0]);
Array Properties and Methods
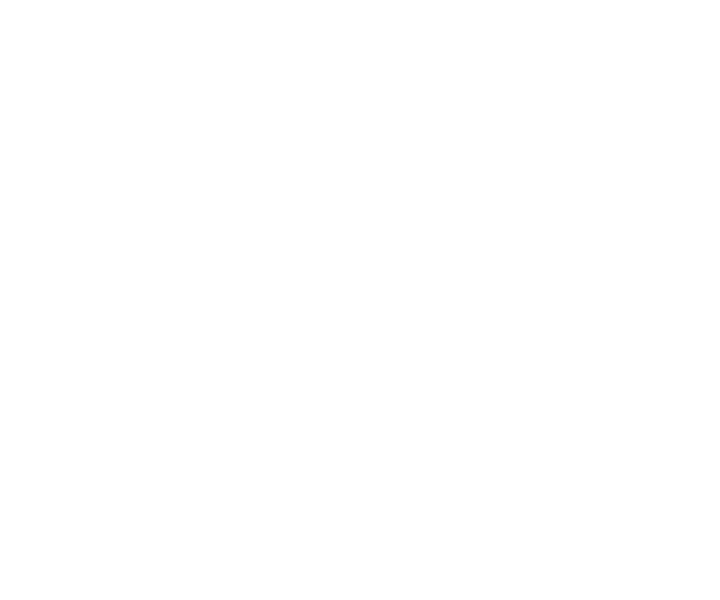
The real power of JavaScript arrays are the built-in array properties and methods:example:-
var x = cars.length; // The length property returns the number of elements
var y = cars.sort(); // The sort() method sorts arrays
Adding Array Elements
The simplest way to add a unique element to an array is using the push() method:
For example:
var fruits = ["Banana", "Orange", "Apple", "Mango"];
fruits.push("Lemon"); // adds a new element (Lemon) to fruits
How to Avoid new Array() in Javascript
There is no need to use JavaScript’s built-in array constructor new Array().
Use []
instead.
These two different statements both create a new empty array named points:
var points = new Array(); // Bad
var points = []; // Good
These two different statements both create a new array containing 6 numbers:
var points = new Array(30, 100, 1, 5, 25, 10); // Bad
var points = [30, 100, 1, 5, 25, 10]; // Good
How to Recognize an Array
A fundamental question is: How do we know if a variable is an array or not?
The problem is that a JavaScript operator typeof returns “object”:
var fruits = ["Banana", "Orange", "Apple", "Mango"];
typeof fruits; // returns object
JavaScript Date Objects
Creating Date Objects
Date objects are designed with the new Date()constructor. There are four ways to create a new date object:
New Date()
New Date(Year, month, day, hours, minutes, seconds, milliseconds)//*Creates date based on specified date and time//
New Date(milliseconds)
new Date(date string)
JavaScript Date Input
There are generally three types of JavaScript date input formats:
Type | Example |
ISO Date | “2015-03-25″ (The International Standard) ” example: var d = new Date(“2015-03-25”); |
Short Date | “03/25/2015” example: var d = new Date(“03/25/2015”); |
Long Date | “Mar 25 2015” or “25 Mar 2015″” example: var d = new Date(“Mar 25 2015”); |
Note: The ISO format follows a strict standard in JavaScript. The other formats are not very well defined and might be browser-specific.
Date.parse()
If you have a confirmed date string, you can use the Date. Parse () method to change it to milliseconds.
Date.parse() returns the number of milliseconds between the date and January 1, 1970:
var msec = Date.parse("March 21, 2012");
document.getElementById("demo").innerHTML = msec;
You can then use the number of milliseconds to convert it to a date object:
var msec = Date.parse("March 21, 2012");
var d = new Date(msec);
document.getElementById("demo").innerHTML = d;
The getFullYear() Method
The getFullYear() method returns the year of a date as a four-digit number:
var d = new Date();
document.getElementById("demo").innerHTML =d.getFullYear();
Set Date Methods
Set Date methods are used for setting a part of a date:
Method | Description |
setDate() | Set the day as a number (1-31) |
setFullYear() | Set the year (optionally month and day) |
setHours() | Set the hour (0-23) |
setMilliseconds() | Set the milliseconds (0-999) |
setMinutes() | Set the minutes (0-59) |
setMonth() | Set the month (0-11) |
setSeconds() | Set the seconds (0-59) |
setTime() | Set the time (milliseconds since January 1, 1970) |
FAQs: in javascript tutorial beginners
To retrieve the month’s day in the js from the Date object, which is the code to select?
var date_obj = new Date(2016,1
,1);Ans: var month_day = date_obj.getDate();
What is true about an array?
Ans: Can have a combination of data types in a single array list
In JavaScript, an object is a container of properties and functions. Properties are identified by ____, and behavior is identified by _____.
Ans: variables, functions
Which is the right way to create an array in JS? a) var myProg = []; b) var myArray = [“C”,”Java”,”C++”,”Python”]; c) var myProg = new Array();
Ans: I, II&III
Can arrays in JavaScript be extended
Ans: Yes, They can.
Above all, we learned about The Object, Date objects, and Array conditions, which are a very important topic of any programming language. The coming article will cover Functions and Closure etc. of this javascript tutorial beginners.
Please stay with us on this journey :).